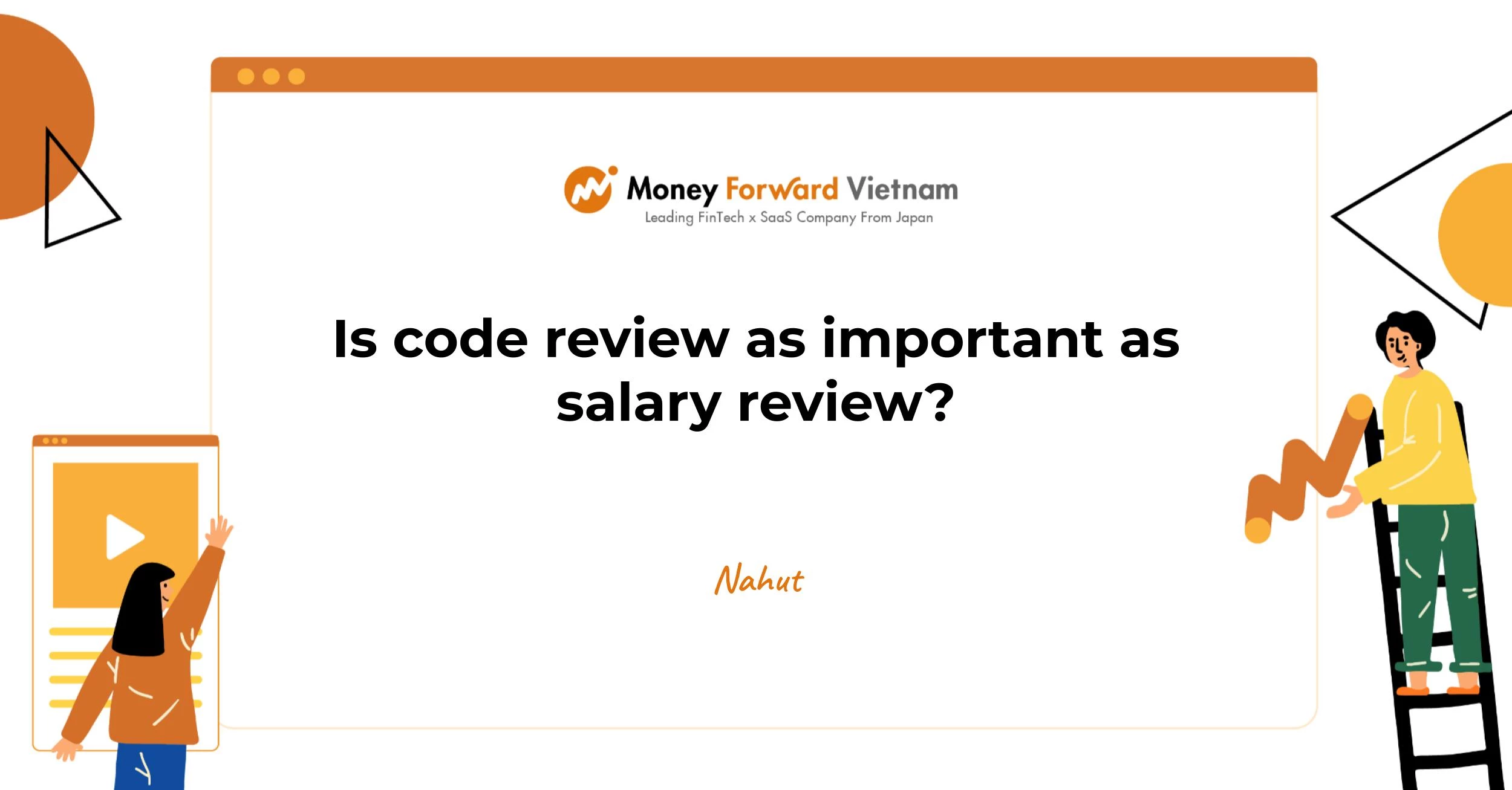
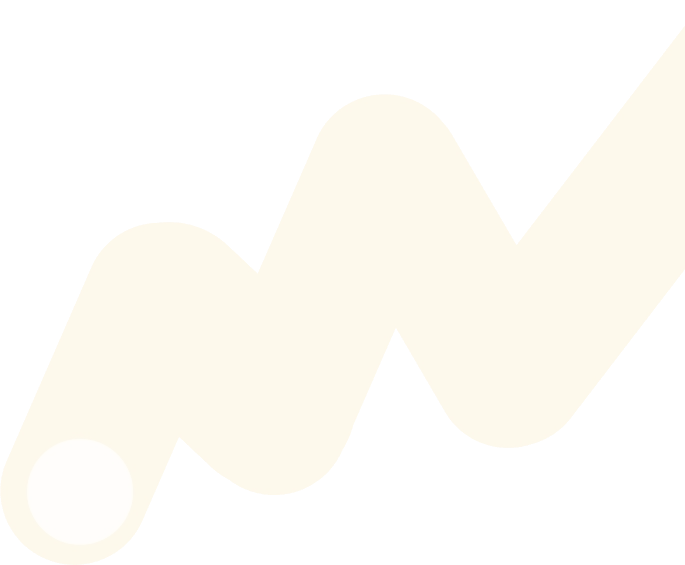
Unlocking Ruby: My Experience with Silver and Gold Certification Exams
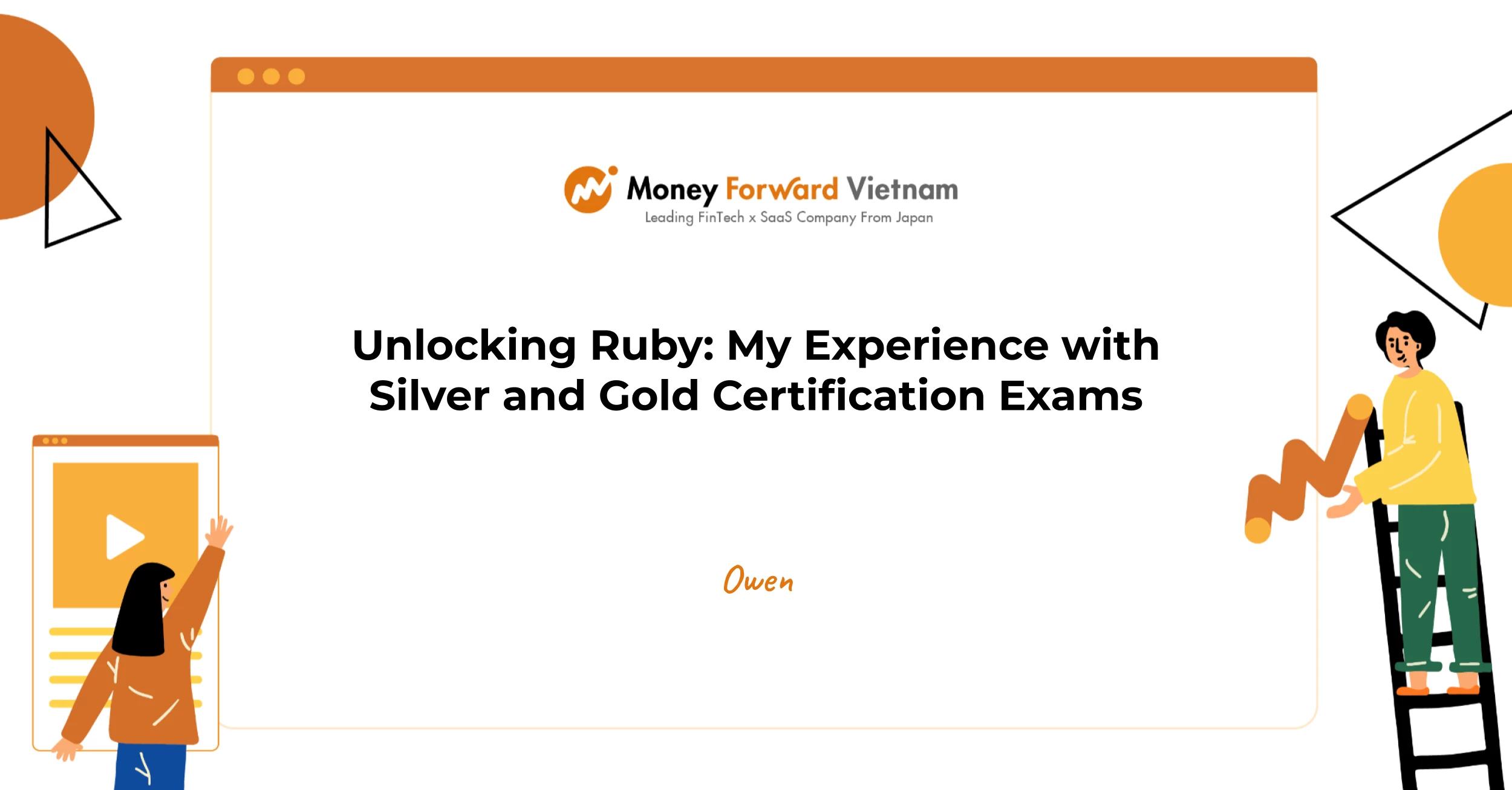
Introduction
Ruby certifications, offered by the Ruby Association, validate a programmer’s proficiency in Ruby programming. These certifications are distinguished into two main levels: Silver and Gold. The Silver certification tests basic Ruby skills and syntax, while the Gold certification is more advanced, testing knowledge of internal mechanisms and libraries.
Choosing the right certification
Understanding the levels: Silver vs Gold
The Silver Level certification is aimed at beginners and ensures that the programmer understands Ruby syntax and basic programming techniques. The Gold Level, on the other hand, is suitable for those who are already familiar with Ruby and want to dive deeper into its object-oriented design and libraries.
Evaluating Your Current Skill Level
Before deciding which exam to start with, assess your current knowledge and experience in Ruby:
If you are new to Ruby, start with the Silver certification.
If you have been working with Ruby daily, particularly with Ruby on Rails, and have a good understanding of the basic and intermediate concepts, consider taking the Gold certification.
Deciding Which Exam to Take First
It's advisable to take the Silver exam first if you are not confident about your advanced Ruby skills. This step-by-step approach helps build a stronger foundation and prepares you better for the Gold exam.
There is an important notice from the Ruby Association:
Candidates must pass both silver and gold exams to be the Ruby Association Certified Ruby Programmer Gold.
In other words, candidates must pass the Silver certification, before they can take the Gold one.
Preparation Strategies
Study Materials
The Ruby documentation ruby-doc.org provides comprehensive material and is a resource you should constantly refer to.
Online courses and tutorials:
Ruby Monk: Free, interactive tutorials to go from beginner to expert.
Viblo practice exams:
RubyEXamination: Free practice exam in Japanese.
A place where you can run Ruby code.
If you prefer using an online editor such as https://try.ruby-lang.org/, make sure the editor is using Ruby version 3.1.x
If you prefer using Ruby on your local machine, you can download it following the instructions here.
Exam details
Exam format
Both exams are computer-based and consist of multiple-choice questions (MCQs). There are a total of 50 questions. Candidates need to answer at least 38 questions correctly to pass the exams.
Silver Exam Specifics
Key topics covered
For the silver level, candidates will be tested on basic syntax, built-in libraries, and basic OOP.
Candidates can check the complete topic list on the exam registration page.
Sample questions
Q1: What is the final output of this command:
p a:1, v:2
Options:
Syntax Error
{:a=>1, :v=>2}
{:a=>1} {:v=>2}
{:v=>2}
Correct answer: 2
Q2: What is the return value of the following code:
a = [3,2,1,3,5]
a.delete(3)
Options:
[2,1,3,5]
[2,1,5]
3
[3,2,1,5]
Correct answer: 3
Q3: Fill in (1) all possible answers to make expected output:
a = [ 4, 5, 6 ]
b = [ 7, 8, 9 ]
______(1)______
p result
=> [[4,7], [5,8], [6,9]]
Options:
result = a.zip b
result = [a,b].transpose
result = [] a.each_with_index do |item, index| result << [item, b[index]] end
result = a.map { |x| [x] } + b.map { |y| [y] }
Correct answer: 1, 2 and 3
Q4: You have a text file named "example.txt" containing multiple lines of text. Each line of the text file contains a single word. Your task is to write a Ruby script to append the string " - modified" to each word in the file and save the changes back to the same file.
Example content of file "example.txt":
apple
banana
cherry
Expected output of file "example.txt":
apple - modified
banana - modified
cherry - modified
Options:
File.open('example.txt', 'r+') do |file| lines = file.readlines lines.map! { |line| line.strip + " - modified\n" } file.puts lines end
File.open('example.txt', 'r+') do |file| lines = file.readlines.map { |line| line.strip + " - modified\n" } file.rewind file.write(lines.join) end
File.open('example.txt', 'a+') do |file| lines = file.readlines.map { |line| line.strip + " - modified" } file.write("\n" + lines.join("\n")) end
File.open('example.txt', 'w+') do |file| original_content = file.read modified_content = original_content.lines.map { |line| line.strip + " - modified\n" } file.write(modified_content.join) end
Correct answer: 2
Option 1 attempts to modify the lines and write them back to the file within the same block. However, it does not properly clear the original content before writing the modified lines back, leading to a jumbled output.
Option 2 correctly reads the lines, modifies them, rewinds the file to the beginning, and writes the new content, effectively replacing the old content.
Opening the file in a+
mode allows for reading and appending but not for truncating the existing content. This method will result in the modified lines being added to the end of the file, rather than modifying the existing lines.
Option 4 opens the file in w+
mode, which truncates all the data before it can be read. This means the original_content
will be empty and nothing will be modified or written back.
Gold Exam Specifics
Key topics covered
For the gold level, candidates will be asked deeper questions related to OOP, advanced syntax. Besides them, Metaprogramming & Singleton Class will also be in the exam scope.Candidates can check the complete topic list on the exam registration page
Sample questions
Q1: What is the output of the puts command below:
module Greeting
def greet
"Hello, "
end
end
module InformalGreeting
include Greeting
def greet
super + "friend!"
end
end
class Person
include Greeting
end
class Friend < Person
include InformalGreeting
end
puts Friend.new.greet
Options:
"Hello, friend!"
"Hello, "
NoMethodError
"friend"
Correct answer: 1
Q2: What is the output of the puts command?
module VerboseGreeting
def self.included(base)
base.alias_method :original_greet, :greet
base.define_method(:greet) do
original_greet + "How are you today?"
end
end
def greet
"Good day, "
end
end
class Person
def greet
"Hello, "
end
end
class Employee < Person
include VerboseGreeting
end
puts Employee.new.greet
Options:
Good day, How are you today?
Hello, How are you today?
Hello,
NoMethodError
Correct answer: 1
When the VerboseGreeting
module is included in the Employee
class, the self.included
hook is triggered. This hook first aliases the existing greet
method from the VerboseGreeting
module to original_greet
. Subsequently, it defines a new greet
method in Employee
that calls original_greet
and appends the string "How are you today?"
. Therefore, when Employee.new.greet
is called, it executes the new greet
method, which calls the aliased original_greet
(returning "Good day, "
) and then appends "How are you today?"
to it.
Q3: What is the output of the code?
def dynamic_modifier(*args, **kwargs)
first = args.shift
last = args.pop
middle_count = args.size
new_kwargs = kwargs.transform_values { |v| v * 2 }
puts "First item: #{first}"
puts "Last item: #{last}"
puts "Remaining items count: #{middle_count}"
puts "New keyword values: #{new_kwargs}"
end
dynamic_modifier(10, 20, 30, 40, rate: 5, speed: 10)
Options:
First item: 10 Last item: 40 Remaining items count: 2 New keyword values: {:rate=>10, :speed=>20}
First item: 10 Last item: 30 Remaining items count: 1 New keyword values: {:rate=> 10, :speed=> 20}
First item: 10 Last item: 40 Remaining items count: 1 New keyword values: {:rate=> 10, :speed=> 20}
First item: 20 Last item: 40 Remaining items count: 2 New keyword values: {:rate=> 5, :speed=> 10}
Correct answer: 1
Q4: What is the output of the code?
info = "The first contact: 123-456-78902. The second one: 322-553-5544. Please call soon."
info.match(/(\d{3})-(\d{3})-(\d{4})/).to_s
Options:
"7890”
"123"
"123-456-7890"
"322-553-5544"
Correct answer: 3
If a match is found, match.to_s
returns the entire matched string, which in this case is "123-456-7890"
. This is because match.to_s
converts the first match object to a string, representing the full sequence captured by the regex.
Q5: What is the output of the code?
module School
CLASS_SIZE = 30
class Classroom
def self.class_size
CLASS_SIZE
end
end
end
module Training
CLASS_SIZE = 20
class Course
include School
def self.class_size
CLASS_SIZE
end
end
end
puts School::Classroom.class_size
puts Training::Course.class_size
Options:
30 30
30 20
20 20
20 30
Correct answer: 2
School::Classroom.class_size
resolves the CLASS_SIZE
within the nearest enclosing scope that defines it, which is within the School
module. Therefore, it correctly outputs 30
.
Training::Course.class_size
might seem tricky because Course
includes the School
module, but Ruby's constant lookup starts in the lexically nearest scope. Since the Training
module itself defines a CLASS_SIZE
, that value (20
) is used before Ruby looks into the included School module. Therefore, it outputs 20
.
On the Day of the Exam
Last-Minute Preparation Tips
Review the main concepts and try to relax. Make sure you know the exam location and what you need to bring with you (ID card, Appointment Confirmation mail).
You may need to prepare a bank card with your signature signed. The supervisor will check that along with your ID card.
What to Expect Physically and Mentally
Be prepared for a few hours at the computer. Take deep breaths, stay hydrated, and keep calm.
Candidates will go through the security check every time they go to the exam room. They need to let all their accessories in the locker, outside the exam room.
Once the candidates click to start the exam, it can not be paused until the time is over. You may need to resolve all your excretory issues before entering the exam room.
The supervisor will go to the exam room to check around every 10 minutes. Just keep doing the exam and ignore them.
Time Management During the Exam
You have about 1.8 minutes to answer each question. Don’t spend too much time on questions you find difficult. Mark them and come back later if you have time.
Challenges Faced
Tricky Questions and How to Handle Them
The question you met in the exam may be trickier than what you have met in practice exams. If a question seems ambiguous, break it down and eliminate the most unlikely answers first.
Stress Management and Mental Blocks
Take short breaks if allowed, and practice deep breathing exercises to maintain your composure.
Post-Exam Reflections
Evaluation of Performance
Reflect on what went well and what didn’t, to better prepare for future certifications.
Quickly remember questions you answer incorrectly and note them right away. You can search for it when you get back home.
Receiving the Results
Certification results are typically available 1 week post-exam. Whatever the outcome, understand that this is a learning process.
Conclusion
The journey to Ruby certification is a challenging yet rewarding one. With the right preparation and mindset, you can excel at both the Silver and Gold exams. Use this guide as a roadmap to your own success. Happy coding!
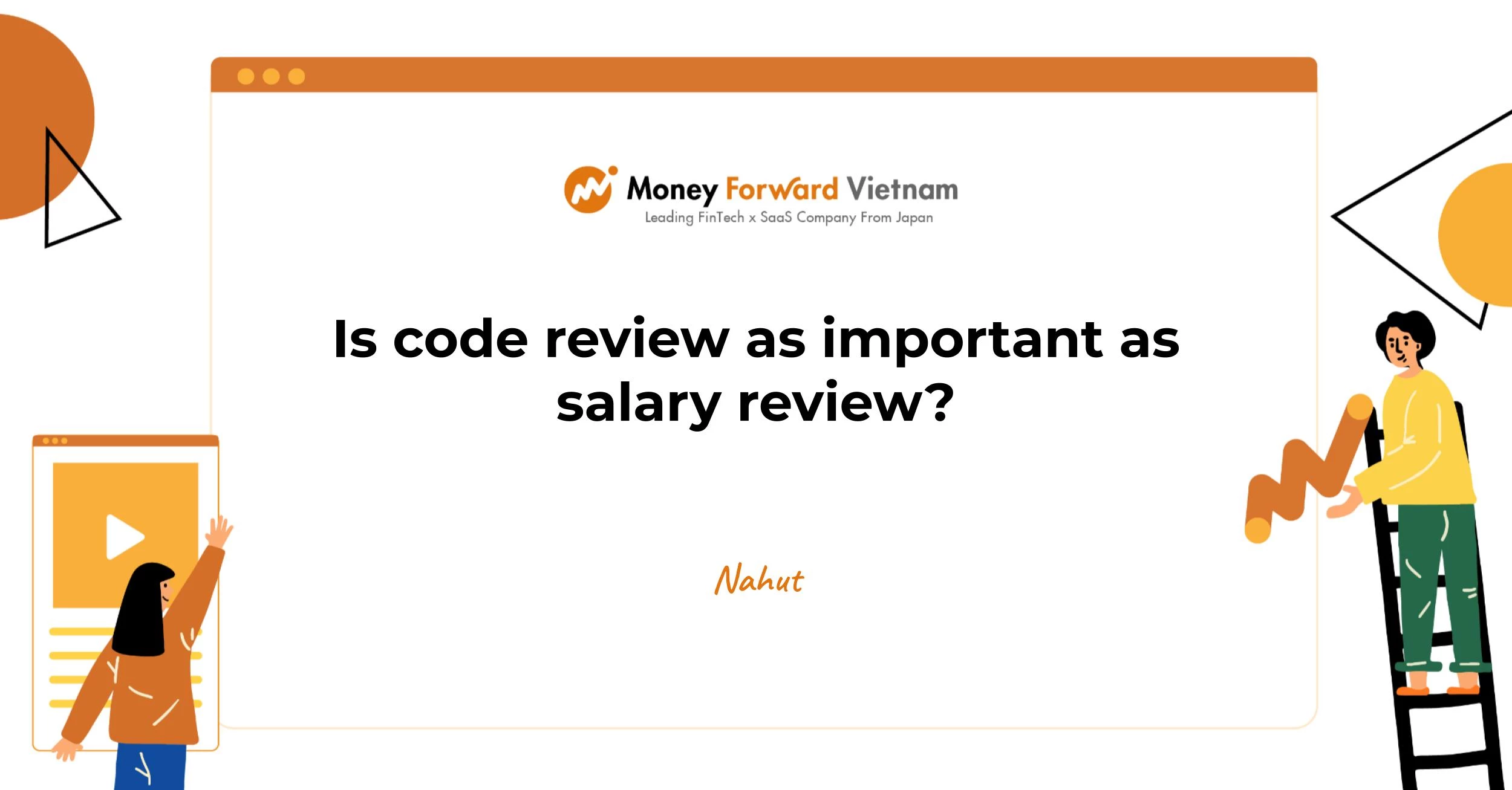
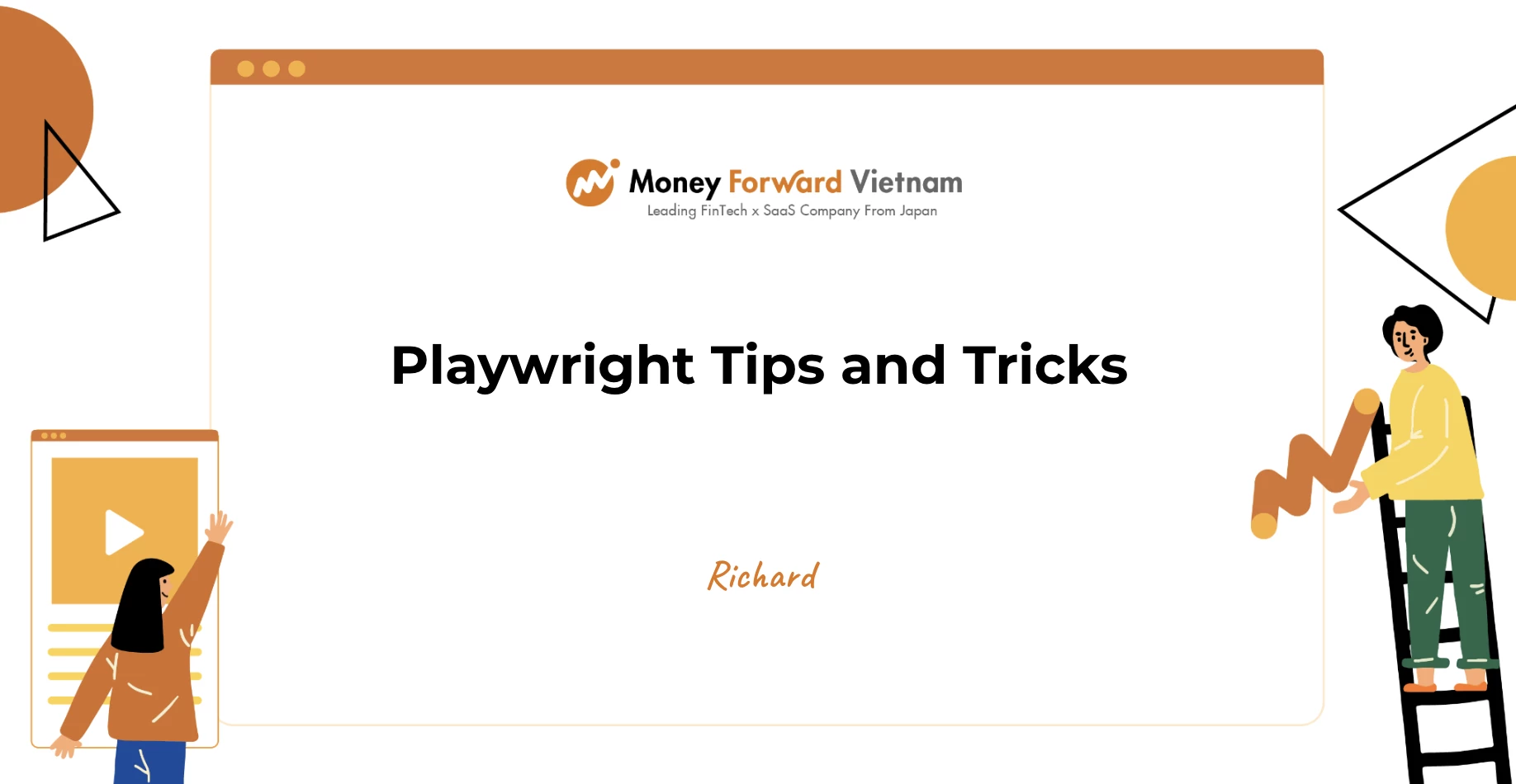
Playwright tips and tricks
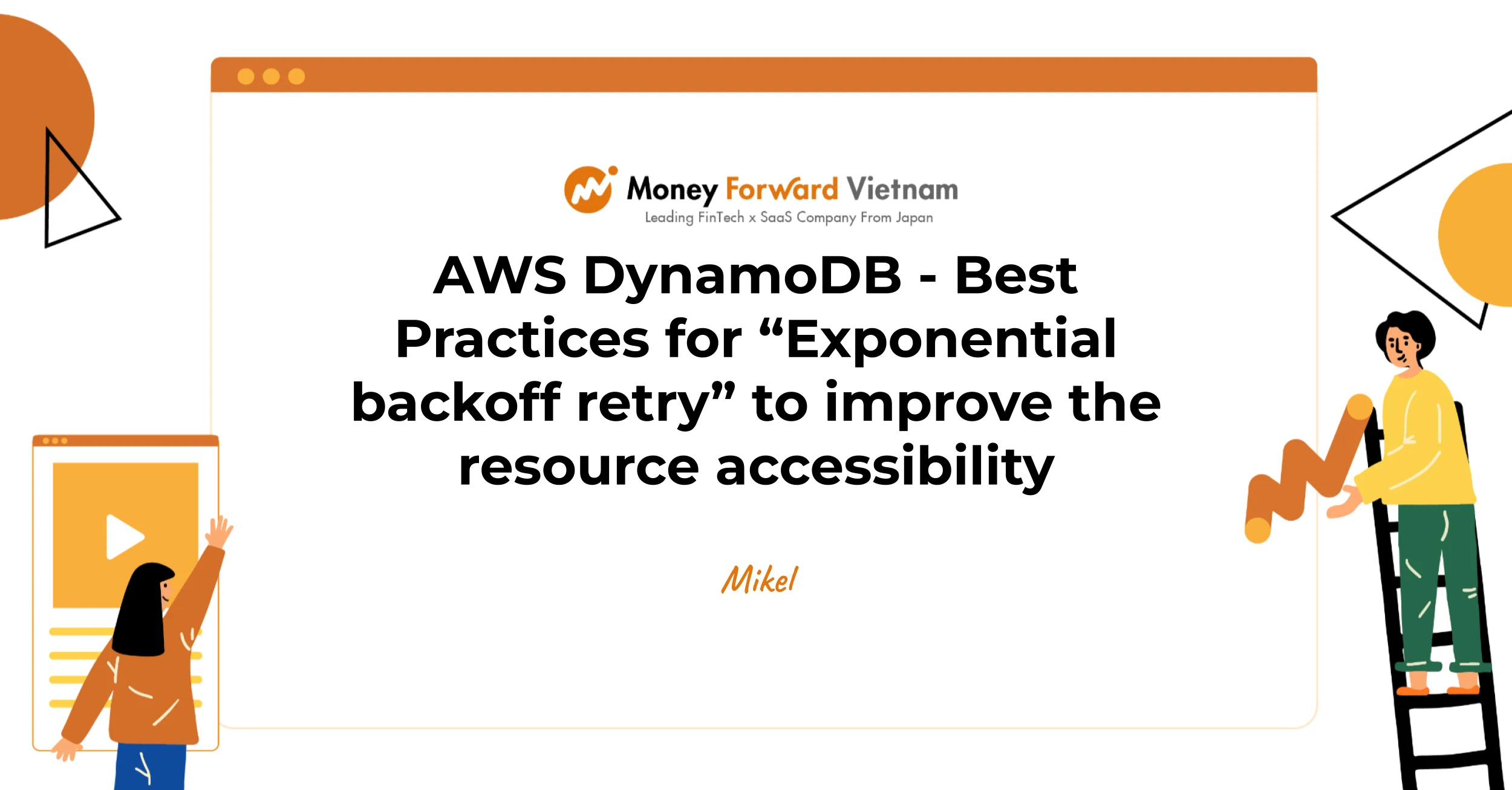