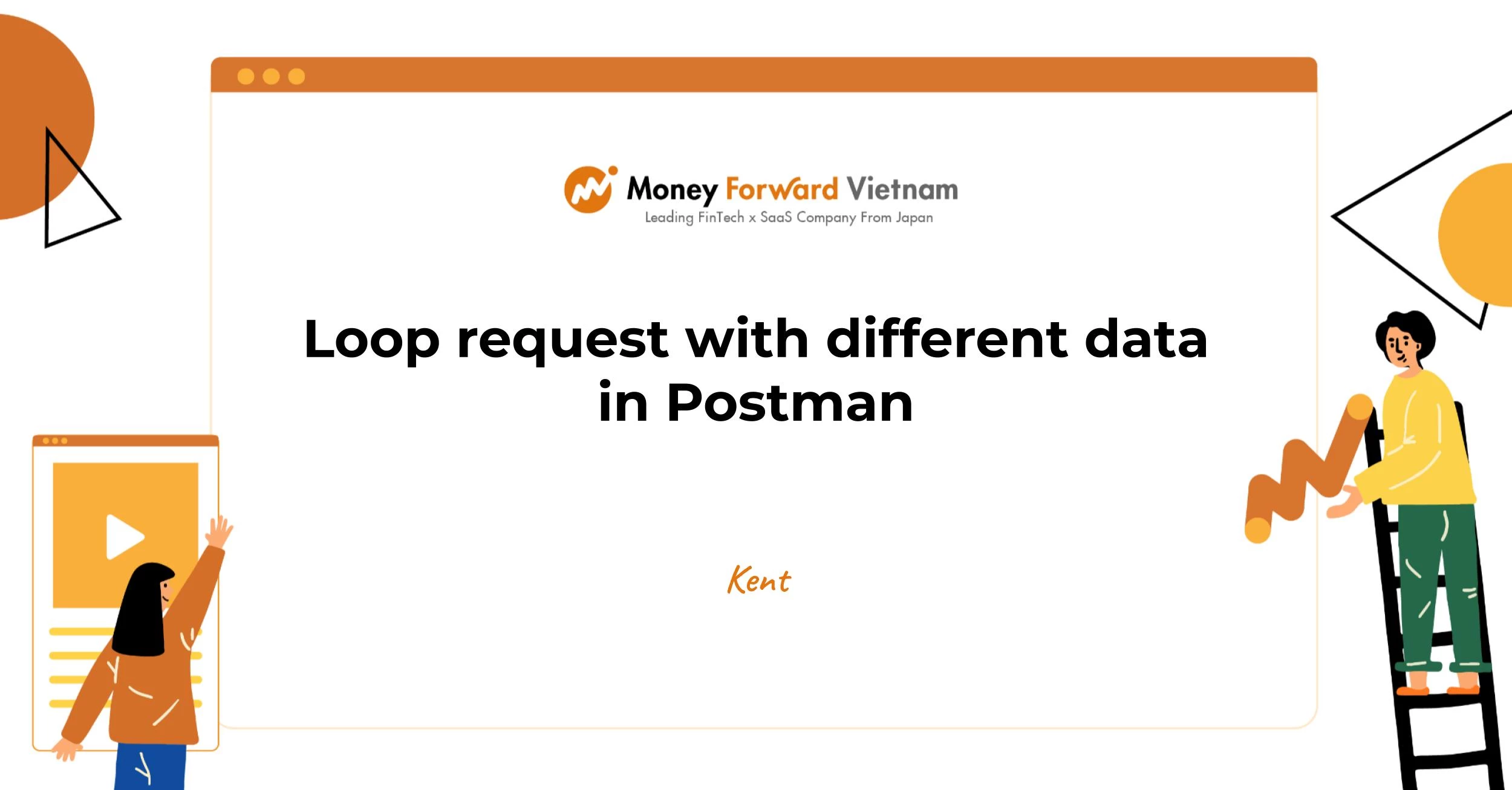
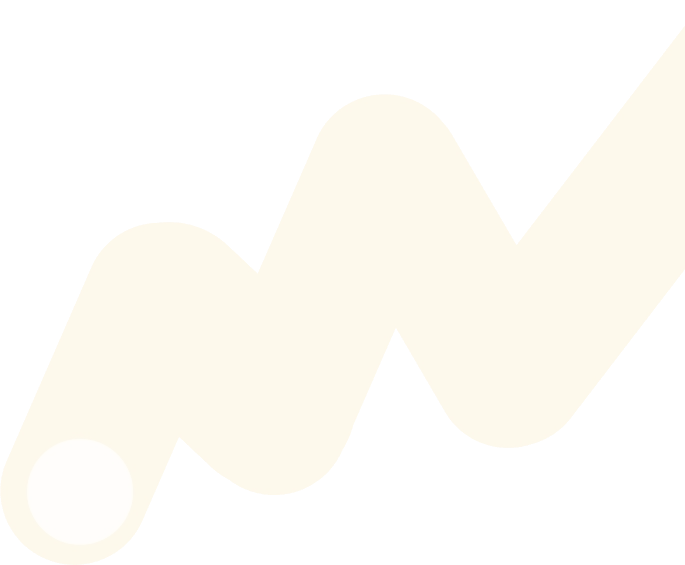
How to create your own gem in Ruby
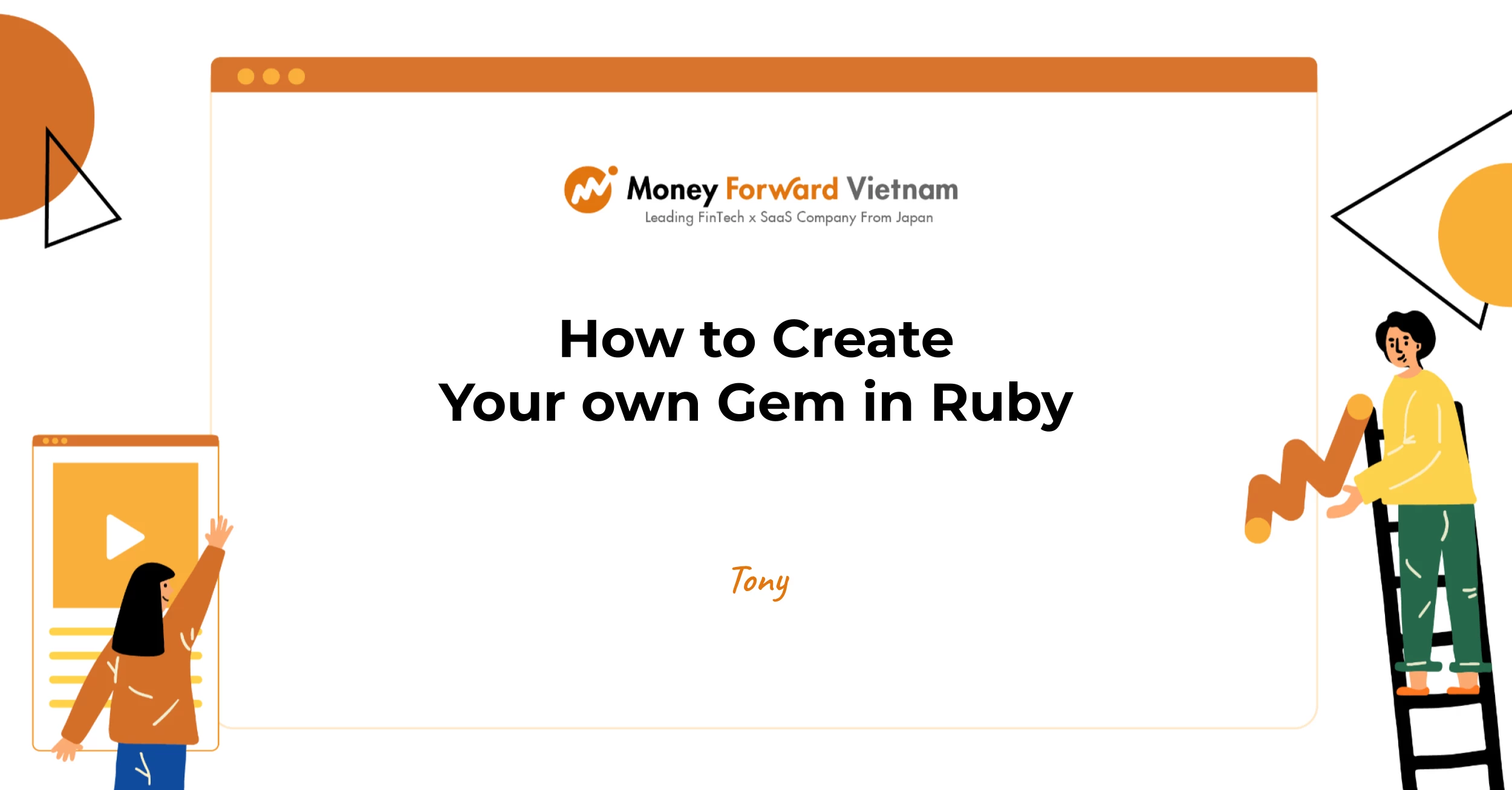
WHAT IS RUBY GEM ?
Gems are open-source Ruby libraries or applications that lend extra utility to other Ruby programs. Each gem is composed of the following:
The source code it was built with (including any tests).
Any documentation with metadata such as the name, version, and platform.
A .gemspec file holding all gem-related metadata.
Gems can be referenced, installed, and published from an online registry called RubyGems.
HOW TO BUILD YOUR OWN GEM ?
Generate Gem Structure
$ gem install bundler
$ bundle gem tony_demo_gem
Change content of file *.gemspec
After you have created a gemspec, you can build a gem from it. Then you can install the generated gem locally to test it out.
$ gem build tony_demo_gem.gemspec
$ gem install ./tony_demo_gem-0.1.0.gem
USING AND DEVELOPMENT
$ gem "tony_demo_gem", git: "https://github.com/tonynguyen-mfv/tony_demo_gem.git"
To install a gem from a local file path, you would use the gem install
command followed by the path to the .gem file. For example:
$ gem "tony_demo_gem", path: "/Users/mfv-computer-0195/Projects/tony_demo_gem"
To install a gem published on RubyGems.org, you would use the gem install command followed by the path to the .gem file. For example:
$ gem "tony_demo_gem"
PUBLISH YOUR GEM
After building your gem, you can use the gem push
command to publish your gem to RubyGems.org. You'll need to have an account on RubyGems.org and be signed in to push your gem. The command would look something like this:
$ gem signin
$ gem push tony_demo_gem-0.1.0.gem
GEM ENGINE CREATION IN RAILS
A Rails engine is a gem that behaves like a miniature Rails application. It allows you to package reusable components, including models, controllers, and views. To generate a new engine, run the following command:
$ rails plugin new tony_demo_plugin --mountable --full
To generate models, controllers, and views run the following command:
$ bin/rails generate scaffold User name:string email:string
Import your gem into rails app:
gem “tony_demo_plugin”, path: “path/to/tony_demo_plugin”
Add the below line to mount your gems route in your rails app.
mount TonyDemoPlugin::Engine => "/tony_demo_plugin"
Add gems migration into your rails app:
$ rails tony_demo_plugin:install:migrations
$ rails db:migrate
Visit http://localhost:3000/tony_demo_plugin/users/
HOW TO OVERRIDE GEM ENGINE
Overriding Models and Controllers
Engine models and controllers can be reopened by the parent application to extend or decorate them.
Overrides may be organized in a dedicated directory app/overrides, ignored by the autoloader, and preloaded in a to_prepare callback:
For example, in order to override the engine model
You just create a file that reopens that class:
For example, in order to override the engine controller:
Overriding Views
You can override this view in the application by simply creating a new file at app/views/*
Other way to Overriding
DEMO
https://github.com/tonynguyen-mfv/tony_demo_gem
https://github.com/tonynguyen-mfv/tony_demo_plugin
https://github.com/tonynguyen-mfv/tony_demo_blog
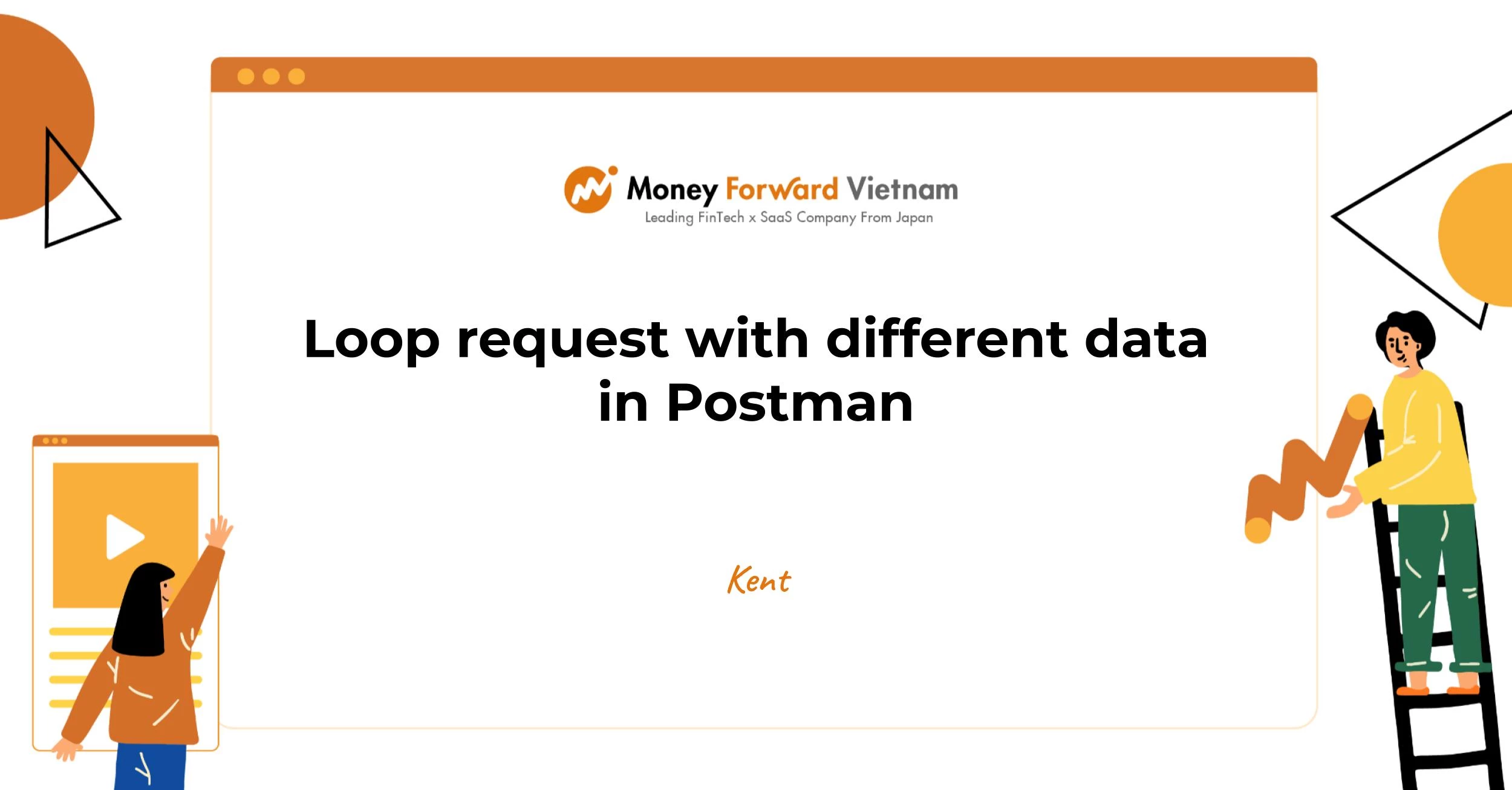
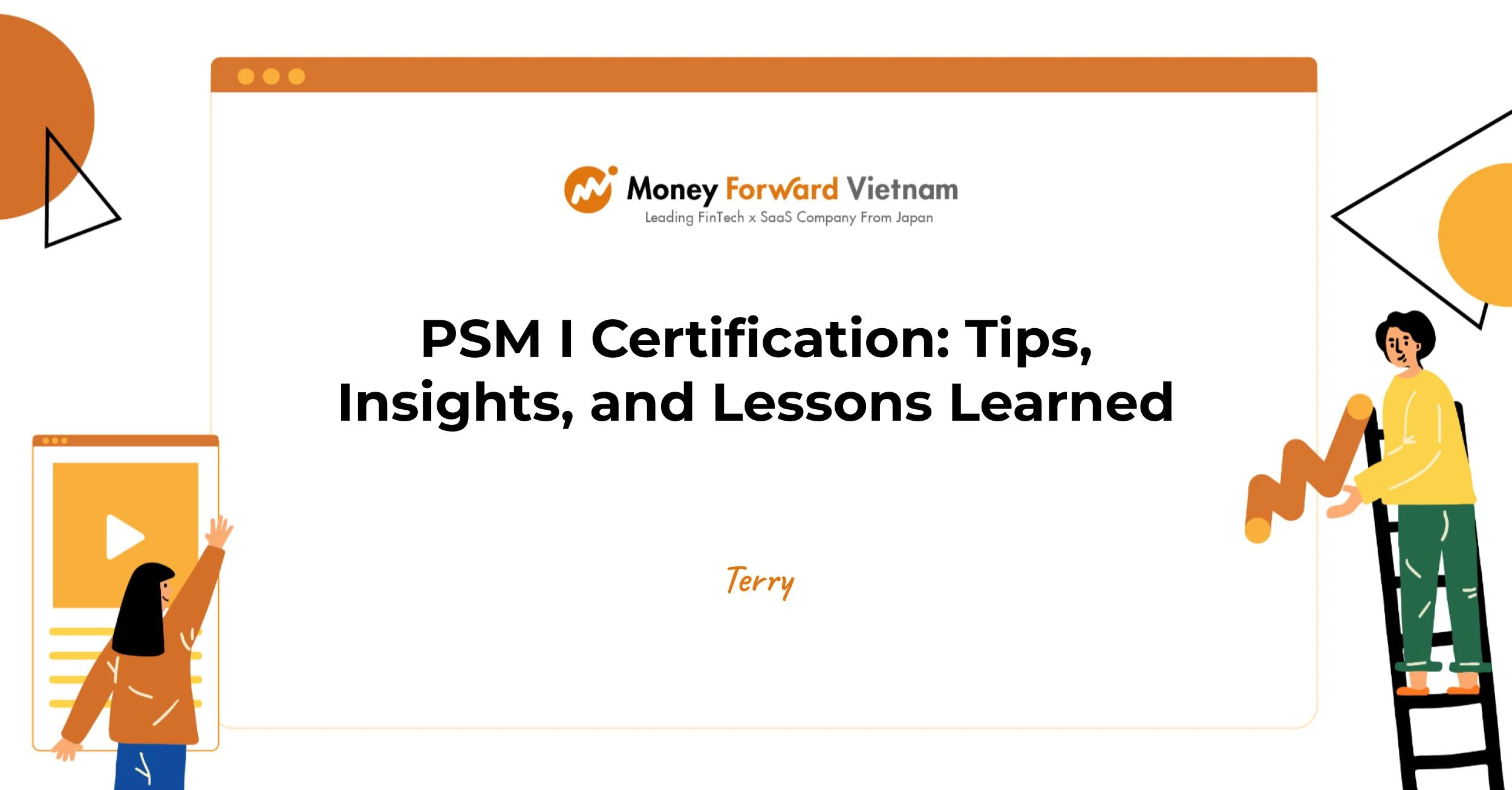
PSM I Certification: Tips, Insights, and Lessons Learned
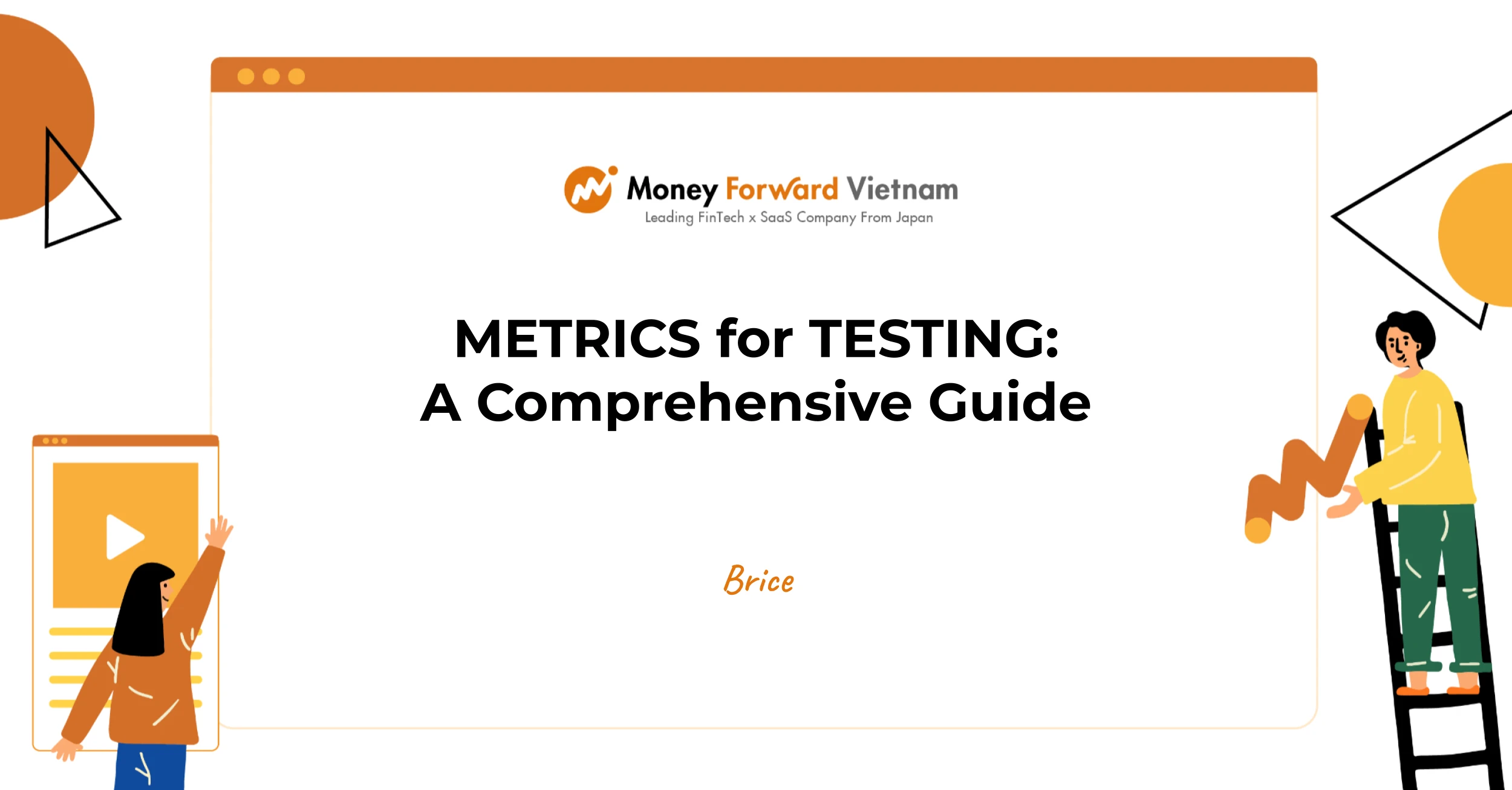